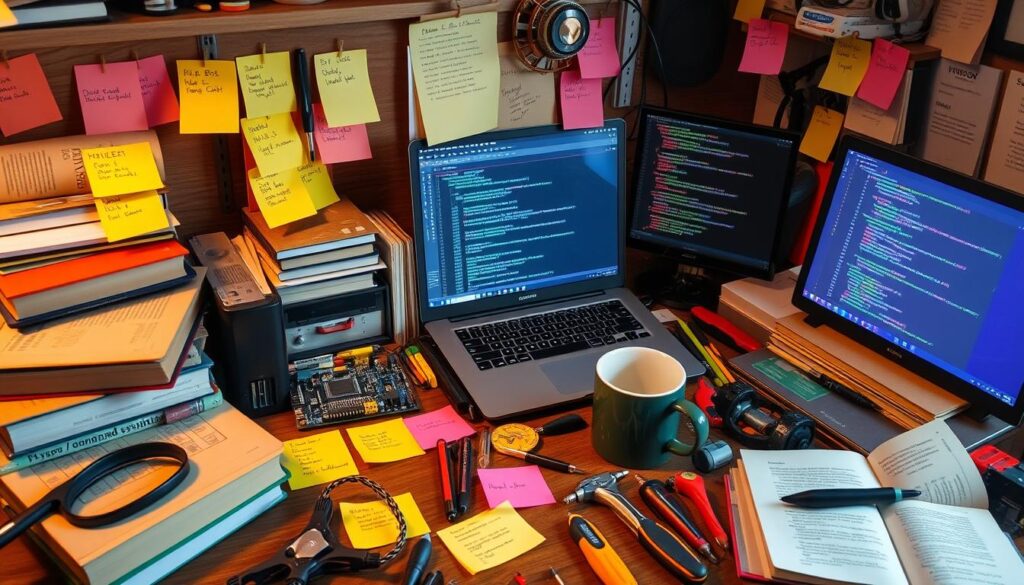
As software developers, we’ve all faced the frustration of code not working. Debugging can seem like a never-ending fight. But it’s a key part of making software work right. Let’s dive into specific ways to make debugging easier1.
In this guide, we’ll share effective strategies and tips to quickly find and fix code errors. We’ll explore everything from rubber ducking to changing how we see bugs. These insights can change how you debug23.
Key Takeaways
- Understand the common types of bugs and how to tackle them effectively.
- Discover the power of rubber ducking – a simple yet effective debugging technique.
- Learn to redefine the nature of a bug, uncovering hidden issues and unexpected behavior.
- Leverage various debugging tools and extensions to enhance your troubleshooting process.
- Adopt a multifaceted approach to debugging, considering different angles and perspectives.
The Essence of Debugging
What is Debugging?
Debugging is finding and fixing errors or bugs in computer programs. These bugs can be syntax errors, runtime errors, or logic errors4. Syntax errors happen when code doesn’t follow the language’s rules, like missing punctuation. Runtime errors occur when code runs but has problems, like dividing by zero4. Logic errors are tricky because they give wrong results but don’t show errors4.
Common Types of Bugs
Even with careful planning, bugs can still appear in software4. Finding bugs can take many tries, especially if they only show up sometimes or in certain places4. Knowing how software has changed over time helps find and fix bugs4. Debugging means finding unexpected system states and figuring out why they happened4.
Testing ideas in debugging is key to avoiding mistakes or biases4. Finding the real cause of software problems takes a lot of work4. Getting others to check your debugging work is important for making sure problems are really fixed4.
“Debugging is the process of finding and resolving defects or problems within a computer program that prevent correct operation.”5
Rubber Ducking: The Art of Talking it Out
Debugging code can be tough, but rubber ducking makes it easier. This method involves talking to a rubber duck about the problem. It’s surprisingly good at finding small errors6.
By talking out loud, programmers can see things they missed before6. It’s like getting a new view on the problem.
The idea behind rubber duck debugging is simple. Talking about your thoughts helps you understand them better7. It makes you think more clearly and spot mistakes7.
Teachers use this idea too. They ask students to explain their answers. This helps students learn on their own7.
It’s best to use an inanimate object for rubber ducking, not a pet7. At work, quietly talk to yourself while debugging. This way, you can find problems without disturbing others7.
Staying calm helps you use verbal debugging effectively6. It helps you find creative solutions to problems6.
Doing relaxing things like showering or jogging can also help6. These activities let your brain relax and come up with new ideas. It’s a way of mental collaboration that helps you avoid quick fixes6.
The art of rubber ducking is very useful for programmers. By talking about the problem, sharing ideas, and checking solutions, you can find the real cause of the issue67.
Moving the Goalposts: Redefining the Bug
As software developers, we often face a constant battle against bugs. These issues seem to appear no matter how hard we try. The real challenge is not just fixing the immediate problem but finding the root cause. This is where bug redefinition comes in, helping us focus and find the real issue.
The Evolution of a Bug
Bugs start with a user-level description, a symptom that users notice right away8. But as we dig deeper, we might find the root cause hidden beneath. This process of bug redefinition is both a mental challenge and key to effective team communication8.
The Process of Redefinition
By redefining the bug, we can focus better and find the real issue8. This ensures the development team is on the same page about the bug8. It’s a dynamic method that needs technical skills, problem-solving, and clear communication8.
The phrase “Moving the Goalposts” perfectly describes how a bug evolves. The initial description gets refined as we investigate further8. This iterative process is key to solving complex software problems8.
“The true genius is the ability to move the goalposts whenever needed to solve a problem.”
Flipping the Direction: Multiple Angles of Attack
Debugging can be tough, especially with complex bugs9. We need to think differently and be more flexible. This means changing how we solve problems.
The usual way to debug is to find the symptom, trace the code, find the problem, and fix it9. But, this method might not work for all problems. That’s when we need to try something new.
The Linear Approach to Debugging
The linear method works well for many bugs9. But, it has its limits. For tough bugs or complex systems, we need to try other ways.
- Reverse engineering the problem to gain a fresh perspective9.
- Changing the environment or testing conditions to expose hidden issues9.
- Challenging our assumptions and preconceptions about the problem at hand9.
By changing our approach, we can find new solutions9.
Collaborative debugging, or “pair debugging,” is very powerful9. Working with someone else can bring new ideas and help solve problems.
“The key to effective debugging is to approach the problem from multiple angles, constantly challenging our assumptions and exploring alternative solutions.”
By being flexible and adaptable, we can improve our debugging skills9. This leads to better software solutions.
⭐️ Tap the exclusive deal link https://temu.to/k/uot8tcxvwum to score top-quality items at ultra-low prices. 🛍️ These unbeatable deals are only available here. Shop now and save big! ⭐️ Directly get exclusive deal in Temu app here: https://app.temu.com/m/mhb5rstagbx
Another surprise for you! Click https://temu.to/k/uag0bn0o0wd to earn with me together🤝!
Disruptive Environments: Exposing Hidden Bugs
Debugging can be tough, especially when bugs are hard to find. But, by adding disruptions to our tests, we can find bugs that might miss us10.
One good way is to make it seem like the network is slow or resources are limited. This shows how our app works when it’s not perfect10. Also, trying different systems, browsers, or hardware can show bugs that only show up in certain setups.
Trying to make things fail or changing the system time can also help. This shows how well our app handles errors or time-based tasks10. By doing these things, we can find bugs that don’t show up in normal tests.
Disruptive Technique | Potential Bugs Uncovered |
---|---|
Network Throttling | Bugs related to degraded performance, network timeouts, or failed API calls |
Resource Limitation | Bugs caused by insufficient memory, CPU, or storage capacity |
Environment Switching | Compatibility issues, platform-specific bugs, and differences in system configurations |
Simulated Failures | Bugs in error handling, exception management, and fault tolerance |
Time Manipulation | Bugs related to time-dependent functionality, such as scheduling, caching, or logging |
Using these methods, we can find bugs that are hard to spot. This makes our apps stronger and more reliable1011.
“Debugging is the art of detecting hidden bugs in your code.” – Unknown
As developers, we need to be ready to try new things in our tests1011. This helps us find bugs that might otherwise stay hidden. It makes our apps more solid and dependable.
Leveraging Debugging Extensions and Tools
As developers, we need the right tools to solve code errors and debugging challenges. We have many tools at our disposal, from browser developer tools to IDE debuggers and profiling utilities. In this section, we’ll look at how to use these tools well to make our development work easier and find hidden problems.
Browser Developer Tools
Browser developer tools, like those in Chrome and Firefox, are crucial for web developers. They give us deep insights into web pages. We can check the Document Object Model (DOM), watch network requests, and set breakpoints in JavaScript code12. These tools have many features and options, helping us solve many problems, from layout issues to performance problems.
IDE Debuggers
Integrated Development Environments (IDEs) have built-in debuggers. These let us step through our code, watch variable values, and check expressions in real-time. These tools give us great insights into our programs, helping us find and fix bugs accurately. Using our IDE’s debugging features, we can work more efficiently and understand our code better.
Profiling and Static Analysis Tools
We can also use profiling and static analysis tools to find performance issues and spot problems in our code without running it13. These tools help us find memory leaks, identify bottlenecks, and catch common coding errors. This lets us fix problems early and prevent them from getting worse later.
By learning about the many debugging tools out there, we can tackle complex code errors and make high-quality software efficiently. From browser tools to IDE debuggers and profiling tools, it’s important to know what each tool does best. Then, we can use them well in our development work.
debugging
Debugging is a key part of making software. It means finding and fixing bugs. We use print statements and version control to help14.
Debugging involves several steps. First, we try to reproduce the bug. Then, we isolate the problem and read error messages14. We also check our assumptions and divide the code into smaller parts15.
Using the “divide and conquer” method helps a lot. It lets us tackle the problem bit by bit. This way, we can find and fix bugs more easily15.
Keeping good documentation is also important. It helps us remember past problems and solutions. Asking for help from others can also reveal new insights15.
Debugging is a skill we need to keep improving16. By mastering techniques like print statements and the divide and conquer method, we make better software141516.
Debugging Technique | Description | Pros | Cons |
---|---|---|---|
Print Statements | Inserting print statements throughout the code to monitor variable values and program flow | Simple to implement, can provide valuable insights into code execution | Can clutter the code, may not be effective for complex bugs |
Version Control | Using version control systems to track changes and rollback to previous working versions | Allows for easy reversal of changes, helps identify when and where a bug was introduced | Requires a good understanding of version control workflows |
Divide and Conquer | Breaking down the problem into smaller, more manageable pieces to isolate the issue | Systematic approach, can uncover the root cause of the bug | Time-consuming, requires a good understanding of the codebase |
Community Support | Seeking help and advice from online programming communities and forums | Fresh perspectives can reveal issues you may have overlooked | Depends on the responsiveness and expertise of the community |
Using these debugging techniques makes finding and fixing bugs easier. This leads to more efficient and reliable software16.
Conclusion
Debugging is key for every software developer. By learning different debugging techniques, we can find and fix errors in our code17. This includes unit testing17, rubber duck debugging17, and print statement debugging17.
Debugging is about finding the problem by trying different things. It’s important to stay calm and methodical. Using tools like debuggers, profilers, and static analysis tools helps us find bugs and improve our work18.
As we get better at debugging, our programs will get better too. We’ll also get better at solving problems as developers18. Being good at debugging is essential for our job. With practice, we can handle even the toughest software challenges1719.
FAQ
What is debugging?
What are the common types of bugs?
What is rubber ducking?
What is the process of redefining a bug?
What are some alternative debugging approaches?
How can disruptive environments help expose hidden bugs?
What are some useful debugging tools and extensions?
What are the key steps in the debugging process?
Source Links
- https://medium.com/javarevisited/debugging-tips-and-tricks-a-comprehensive-guide-8d84a58ca9f2 – Debugging Tips and Tricks: A Comprehensive Guide
- https://dev.to/saloman_james/debugging-101-how-to-find-and-fix-programming-errors-1d14 – Debugging 101: How to Find and Fix Programming Errors
- https://medium.com/upperlinecode/debugging-is-fun-sometimes-tips-on-dealing-with-errors-in-your-code-b99fad94ad3d – Debugging is Fun (Sometimes): Tips on Dealing with Errors in Your Code
- https://blog.scitools.com/the-science-of-debugging/ – The Science of Debugging – SciTools Blog
- https://stackoverflow.com/questions/728456/standard-methods-of-debugging – Standard methods of debugging
- https://dev.to/zenstack/why-do-rubber-ducks-work-27ia – Why Do Rubber Ducks Work?
- https://medium.com/@arnoldcsubastil/the-psychology-behind-rubber-duck-debugging-f19141c70060 – The Psychology Behind Rubber Duck Debugging
- https://www.codebrawl.com/debugging-large-scale-applications/ – Effective Strategies for Debugging Large-Scale Applications
- https://discussions.unity.com/t/2d-melee-attack-point-cant-flip/882998 – 2D Melee attack point can’t flip?
- https://www.linkedin.com/pulse/mastering-android-app-debugging-5-tips-from-experts-asiq-m-vqajc – Mastering Android App Debugging; 5 Tips from Experts
- https://fastercapital.com/content/Software-Bugs–Uncovering-the-Hidden-Glitches–A-Deep-Dive-into-BugsIndex.html – Software Bugs: Uncovering the Hidden Glitches: A Deep Dive into BugsIndex – FasterCapital
- https://developer.chrome.com/docs/extensions/mv2/tutorials/debugging – Debugging extensions | Manifest V2 | Chrome for Developers
- https://resources.esri.ca/getting-technical/debugging-your-web-gis-applications-leveraging-your-browser-s-developer-tools – Debugging your web GIS applications – Leveraging your browser’s developer tools
- https://learn.microsoft.com/en-us/visualstudio/debugger/what-is-debugging?view=vs-2022 – What is debugging and a debugger? – Visual Studio (Windows)
- https://www.techtarget.com/searchsoftwarequality/definition/debugging – What is debugging?
- https://goodspeed.studio/glossary/what-is-debugging-debugging-explained – What is Debugging? Debugging Explained I No Code Glossary
- https://medium.com/@pixelprofits/effective-debugging-strategies-and-tools-for-software-developers-28094484260b – Effective Debugging: Strategies and Tools for Software Developers
- https://sabio.la/blog/resources/troubleshoot-like-a-pro-the-art-of-debugging-in-programming – Troubleshoot Like a Pro: The Art of Debugging in Programming
- https://aws.amazon.com/what-is/debugging/ – What is Debugging? – Debugging Explained – AWS
- https://www.youtube.com/shorts/wq3BA9oUU7E