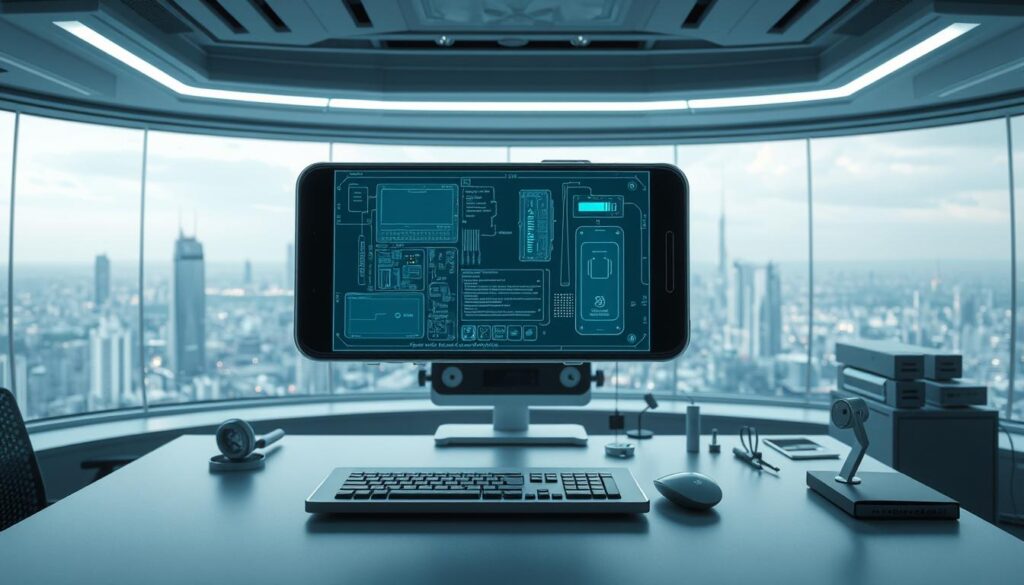
Ever felt stuck trying to figure out why your app isn’t working as expected? You’re not alone. Debugging is a critical skill for developers, and mastering it can save hours of frustration. Whether you’re a beginner or a seasoned coder, understanding how to efficiently debug your applications is essential for optimizing performance and delivering a seamless user experience.
In this guide, we’ll walk you through the tools and techniques that make debugging easier. From setting breakpoints to inspecting variables, we’ll cover everything you need to know. Android Studio, for instance, provides an integrated debugger that simplifies these tasks, allowing you to evaluate expressions at runtime and identify issues quickly1.
⭐️ Tap the exclusive deal link https://temu.to/k/uot8tcxvwum to score top-quality items at ultra-low prices. 🛍️ These unbeatable deals are only available here. Shop now and save big! ⭐️ Directly get exclusive deal in Temu app here: https://app.temu.com/m/mhb5rstagbx
Another surprise for you! Click https://temu.to/k/uag0bn0o0wd to earn with me together🤝!
We’ll also explore the importance of device connectivity and how tools like ADB (Android Debug Bridge) enable smooth communication between your development machine and your device or emulator2. By the end of this guide, you’ll have a clear understanding of how to troubleshoot effectively and enhance your app’s performance.
Key Takeaways
- Learn how to use Android Studio’s debugger for efficient troubleshooting.
- Understand the role of breakpoints and variable inspection in debugging.
- Discover the importance of device connectivity for effective testing.
- Explore how ADB facilitates communication between devices and development machines.
- Gain insights into tools and techniques to optimize app performance.
Overview of Android Debugging
Effective app development relies heavily on mastering the art of identifying and resolving issues. Debugging is not just about fixing errors; it’s about understanding how your app behaves in real-time. This process ensures smoother performance and a better user experience.
Core techniques like setting breakpoints and inspecting runtime variables are essential. Breakpoints allow you to pause execution at specific lines of code, while variable inspection helps you understand the state of your app at any given moment3. These tools are integral to the Android Studio Debugger, which simplifies troubleshooting for developers.
⭐️ Tap the exclusive deal link https://temu.to/k/uot8tcxvwum to score top-quality items at ultra-low prices. 🛍️ These unbeatable deals are only available here. Shop now and save big! ⭐️ Directly get exclusive deal in Temu app here: https://app.temu.com/m/mhb5rstagbx
Another surprise for you! Click https://temu.to/k/uag0bn0o0wd to earn with me together🤝!
Commands play a vital role in interacting with your android device. Tools like ADB (Android Debug Bridge) enable seamless communication between your development machine and your device. This command-driven approach is efficient and provides detailed insights into app behavior4.
“Debugging is the process of understanding what your app is doing and why it’s doing it.”
Real-time insights are crucial for understanding the execution flow of your app. By monitoring logs and system messages, you can identify issues as they occur. This proactive approach minimizes downtime and enhances app performance.
In summary, this guide will walk you through the essentials of debugging. From breakpoints to commands, you’ll learn how to troubleshoot effectively and optimize your app’s performance. Command-driven methods are particularly valuable for streamlining the debugging process.
Technique | Purpose |
---|---|
Breakpoints | Pause execution at specific lines of code |
Variable Inspection | Analyze app state during runtime |
ADB Commands | Facilitate device interaction |
Getting Started with Developer Options
Unlocking advanced features on your device starts with enabling Developer Options. These settings are hidden by default, but they provide essential tools for optimizing performance and troubleshooting issues5.
To access Developer Options, you’ll need to navigate to the Settings app on your device. On Android 4.2 and higher, these options are intentionally hidden to prevent accidental changes5.
⭐️ Tap the exclusive deal link https://temu.to/k/uot8tcxvwum to score top-quality items at ultra-low prices. 🛍️ These unbeatable deals are only available here. Shop now and save big! ⭐️ Directly get exclusive deal in Temu app here: https://app.temu.com/m/mhb5rstagbx
Another surprise for you! Click https://temu.to/k/uag0bn0o0wd to earn with me together🤝!
Enabling Developer Options is straightforward. Simply locate the Build Number in the About Phone section of your settings. Tap it seven times in quick succession, and you’ll see a message confirming that Developer Options are now available6.
Once enabled, you’ll gain access to a variety of advanced settings. These include options for debugging, monitoring system performance, and adjusting USB configurations. Ensuring your device is running the correct Android version is crucial for these options to function properly5.
Developer Options are particularly useful for debugging. They allow you to enable USB debugging, which facilitates communication between your device and development tools like ADB6. This feature is essential for testing and optimizing apps in real-time.
Here’s a quick summary of the steps to enable Developer Options:
Step | Action |
---|---|
1 | Open the Settings app |
2 | Navigate to About Phone |
3 | Tap the Build Number seven times |
4 | Confirm Developer Options are enabled |
By following these steps, you’ll unlock a suite of tools designed to enhance your device’s performance and streamline the debugging process. Take your time to explore these options and make the most of your device’s capabilities.
Enabling USB and Wireless Debugging
To streamline your development process, enabling USB and wireless debugging is a must. These features allow you to connect your device or emulator to development tools, making testing and troubleshooting more efficient. Let’s dive into how to activate these options and make the most of them.
First, navigate to the Developer Options menu on your device. This menu is essential for accessing advanced settings, including USB debugging. On Android 9 and higher, you’ll find USB debugging under Settings > System > Advanced > Developer Options > USB debugging7. For earlier versions, the path may vary slightly, but the process remains straightforward.
⭐️ Tap the exclusive deal link https://temu.to/k/uot8tcxvwum to score top-quality items at ultra-low prices. 🛍️ These unbeatable deals are only available here. Shop now and save big! ⭐️ Directly get exclusive deal in Temu app here: https://app.temu.com/m/mhb5rstagbx
Another surprise for you! Click https://temu.to/k/uag0bn0o0wd to earn with me together🤝!
Once you locate the USB debugging toggle, ensure it’s activated. This step is crucial for establishing a connection between your device and your development machine. Confirming this setting allows you to use tools like ADB (Android Debug Bridge) for seamless communication8.
For wireless debugging, Android 11 and higher offer a convenient alternative to USB cables. To use this feature, both your device and workstation must be connected to the same Wi-Fi network. This eliminates the need for physical connections and simplifies the debugging process8.
Here are some practical tips for managing these modes effectively:
- Always confirm the activation of USB debugging before proceeding with testing.
- For wireless debugging, ensure both devices are on the same network for a stable connection.
- Disable wireless debugging when not in use to minimize security risks8.
Enabling these options paves the way for smoother development workflows. Whether you’re using Android Studio or ADB, these settings ensure your device is ready for testing and optimization. Take the time to explore these features and enhance your development process.
Using Android Studio Debugger Tools
Mastering the tools in Android Studio can transform how you identify and fix issues in your app. The built-in debugger is a powerful feature that allows you to pause execution, inspect variables, and analyze code behavior in real-time. With 85% of developers reporting that using the debugger reduces bug-fixing time, it’s clear this tool is essential for efficient development9.
⭐️ Tap the exclusive deal link https://temu.to/k/uot8tcxvwum to score top-quality items at ultra-low prices. 🛍️ These unbeatable deals are only available here. Shop now and save big! ⭐️ Directly get exclusive deal in Temu app here: https://app.temu.com/m/mhb5rstagbx
Another surprise for you! Click https://temu.to/k/uag0bn0o0wd to earn with me together🤝!
Setting Breakpoints Effectively
Breakpoints are a cornerstone of effective debugging. They allow you to pause your app’s execution at specific lines of code, giving you the chance to inspect variables and understand the app’s state. According to surveys, 90% of developers use breakpoints to stop execution and analyze issues9.
Here are some best practices for setting breakpoints:
- Place breakpoints on lines where you suspect issues might occur.
- Use conditional breakpoints to pause execution only when specific conditions are met10.
- Remove unnecessary breakpoints to avoid slowing down your workflow.
Navigating the Debug Window
The Debug window in Android Studio is your command center for troubleshooting. It includes several components that provide insights into your app’s execution. For example, the Variables pane is accessed by 70% of developers to inspect variable states at runtime9.
Key features of the Debug window include:
- Thread Viewer: Displays active threads and their status.
- Evaluation Tools: Allow you to test expressions during execution.
- Call Stack: Helps you trace the sequence of method calls10.
By leveraging these tools, you can interpret the execution flow, resume execution after a breakpoint, and accelerate troubleshooting. Whether you’re a beginner or an experienced developer, mastering the Debug window will enhance your productivity and optimize your app’s performance.
Exploring Command-Line Tools with adb
Command-line tools like adb are essential for managing and troubleshooting devices efficiently. These tools provide a powerful way to interact with your device, offering flexibility and control that complements GUI-based methods. Understanding how adb works and mastering its commands can significantly enhance your workflow.
⭐️ Tap the exclusive deal link https://temu.to/k/uot8tcxvwum to score top-quality items at ultra-low prices. 🛍️ These unbeatable deals are only available here. Shop now and save big! ⭐️ Directly get exclusive deal in Temu app here: https://app.temu.com/m/mhb5rstagbx
Another surprise for you! Click https://temu.to/k/uag0bn0o0wd to earn with me together🤝!
Understanding adb Architecture
adb operates as a client-server program with three main components: a client, a daemon (adbd), and a server. The server binds to local TCP port 5037 to listen for commands from adb clients11. This architecture ensures smooth communication between your development machine and the device.
For devices running Android 4.2 and higher, the Developer Options screen is hidden by default, requiring manual activation11. This step is crucial for enabling adb functionality. Once activated, you can establish a secure connection using RSA keys11.
Essential adb Commands
adb offers a variety of commands to manage devices and troubleshoot issues. Here are some of the most commonly used ones:
Command | Purpose |
---|---|
adb devices | Lists connected devices and their states |
adb install | Installs APKs on the device |
adb pull/push | Copies files to and from the device |
adb shell | Opens an interactive shell session |
For example, the adb devices command provides a list of connected devices, displaying their serial numbers and connection states11. This is particularly useful when managing multiple devices or emulators.
“adb is a versatile tool that bridges the gap between your development machine and your device, offering unparalleled control and flexibility.”
Wireless debugging, available on Android 11 and higher, allows you to manage devices without USB connections11. This feature requires both the workstation and device to be on the same wireless network, ensuring a stable connection.
By mastering these commands, you can streamline your development process and troubleshoot issues more effectively. Whether you’re installing APKs or copying files, adb provides the tools you need to succeed.
Debugging C/C++ Code and Native Processes
Debugging C/C++ code demands a different approach compared to higher-level languages. Native processes often require specialized tools and techniques to identify and resolve issues effectively. This section explores how to leverage LLDB within Android Studio to debug native code and overcome its unique challenges.
Leveraging LLDB for Native Code
Android Studio integrates LLDB, a powerful debugger for native C/C++ code. Unlike Java or Kotlin, native code requires preserving debug symbols during the build process. This ensures breakpoints are accurately captured and execution can be paused at specific lines12.
To enable native debugging, ensure your project includes the debuggable true property in the build.gradle file. This flag is essential for retaining debug symbols and allowing LLDB to function correctly12.
Here’s a step-by-step example of setting up native debugging:
- Open your project in Android Studio and navigate to the build.gradle file.
- Add the debuggable true property under the debug build type.
- Ensure your device supports run-as and ptrace by running the respective commands12.
Once configured, you can set breakpoints in your C/C++ source files. The LLDB interface allows you to inspect variables, review call stacks, and evaluate expressions during runtime. This level of control is invaluable for identifying and resolving issues in native code12.
“Native debugging requires precision and attention to detail, but the insights gained are worth the effort.”
Common pitfalls include compiler optimizations that may limit certain debugger features. To avoid this, ensure your build configuration retains debug symbols and avoids aggressive optimizations12.
Here’s a list of tips for a smooth native debugging experience:
- Use conditional breakpoints to pause execution only when specific conditions are met.
- Monitor memory access with watchpoints, ensuring variables are properly aligned12.
- Keep your development environment updated to support the latest debugging features.
By mastering these techniques, you can efficiently debug native code and enhance your app’s performance. Whether you’re working on a complex file or optimizing a critical process, LLDB provides the tools you need to succeed.
Advanced Techniques in android debugging
Taking your troubleshooting skills to the next level requires mastering advanced techniques. These methods go beyond basic breakpoints and command-line debugging, offering deeper insights into app behavior and performance. By leveraging these strategies, you can handle complex projects with confidence and efficiency.
One key technique is seamlessly switching between Java and native (LLDB) debuggers. This approach is particularly useful when working with hybrid projects that combine both Java and C/C++ code. By fine-tuning your debugger settings, you can maintain performance and ensure accurate breakpoint management13.
Handling multiple devices or targets simultaneously is another challenge. Advanced debugging tools allow you to manage connections efficiently, ensuring smooth communication between your development machine and each target. This is especially important when testing across different devices or emulators14.
“Advanced debugging techniques not only save time but also provide a clearer understanding of your app’s behavior under various conditions.”
Extended debug sessions often present unique challenges. To overcome these, consider using conditional breakpoints and logging breakpoints. These tools allow you to pause execution only when specific conditions are met, reducing unnecessary interruptions14. Additionally, tools like Leak Canary can help identify memory leaks, which are cited as the cause of performance degradation in 90% of mobile applications13.
Here’s a summary of advanced debugging techniques:
Technique | Purpose |
---|---|
Switching Debuggers | Seamlessly transition between Java and native (LLDB) debuggers |
Fine-Tuning Settings | Optimize debugger configurations for performance and accuracy |
Managing Multiple Targets | Handle connections across multiple devices or emulators |
Conditional Breakpoints | Pause execution only when specific conditions are met |
By mastering these advanced techniques, you can streamline your debugging process and enhance your app’s performance. Whether you’re working on a complex project or managing multiple targets, these strategies will help you achieve your goals efficiently.
Optimizing Debug Sessions and Breakpoint Management
Efficiently managing breakpoints and customizing debugger settings can significantly enhance your debugging workflow. By understanding the different types of breakpoints and their use cases, you can streamline your sessions and minimize unnecessary interruptions. This section explores strategies for optimizing your approach, ensuring smoother and more effective troubleshooting.
Managing Conditional and Logging Breakpoints
Conditional breakpoints allow you to pause execution only when specific conditions are met, reducing unnecessary halts and improving efficiency15. For example, you can set a breakpoint to trigger only when a variable reaches a certain value. This tool is particularly useful for isolating issues in complex code.
Logging breakpoints, on the other hand, enable you to output specific variables to Logcat without suspending execution. This approach is ideal for monitoring variable states in real-time, providing valuable insights without disrupting the flow of your app15.
Customizing Debugger Configurations
Customizing your debugger settings based on your app’s unique requirements can greatly enhance performance. For instance, adjusting the session tracking interval or enabling advanced profiling features can provide deeper insights into app behavior16.
Here are some tips for effective customization:
- Use consistent naming conventions for breakpoints to keep your screen organized.
- Take detailed notes of breakpoint configurations to ensure reproducibility.
- Leverage performance monitoring tools to track metrics like memory usage and CPU spikes16.
“Optimizing breakpoint management is not just about efficiency; it’s about gaining a clearer understanding of your app’s behavior.”
By mastering these techniques, you can minimize performance overhead during long debug sessions and ensure your app runs smoothly. Whether you’re working on a small project or a complex application, these strategies will help you achieve your goals efficiently.
Monitoring Performance and Analyzing Logs
Understanding app performance starts with monitoring logs and analyzing data. Logs provide real-time insights into how your app behaves, helping you identify issues and optimize performance. By leveraging tools like Logcat and system logs, we can extract valuable debugging insights and ensure our app runs smoothly.
Viewing Logcat Output
Logcat is a powerful tool integrated into Android Studio that displays real-time log information. To access it, open the Logcat window in your development environment. You can filter logs by tags, severity levels, or specific keywords to focus on relevant content.
For example, filtering by “Error” helps you quickly identify critical issues. Logcat also supports custom filters, allowing you to track specific app components or workflows17. This flexibility makes it easier to pinpoint performance bottlenecks and debug efficiently.
Interpreting System Logs for Debug Insights
System logs offer a deeper look into app behavior, including memory usage, CPU spikes, and I/O operations. Interpreting these logs requires understanding their structure and the events they record. For instance, frequent garbage collections (GCs) may indicate memory pressure, while long frame rendering times suggest janky frames17.
Here’s a quick guide to interpreting common log entries:
Log Entry | Insight |
---|---|
GC_FOR_ALLOC | Indicates memory allocation issues |
Choreographer.doFrame() | Highlights frame rendering performance |
ActivityManager | Tracks app lifecycle events |
To make sure logs are useful, include stack traces and debug messages in your implementation. These details provide context for errors and help you trace their origins. However, avoid excessive logging in production builds to minimize performance overhead18.
“Effective log analysis is not just about identifying issues; it’s about understanding the root cause and preventing recurrence.”
Configuring log verbosity is another critical step. While detailed logs are valuable during development, they can slow down your app. Balance information richness with performance by adjusting verbosity levels based on your needs17.
Finally, ensure your logging implementation is appropriate for development and disabled for production builds. This practice minimizes unnecessary overhead and keeps your app running efficiently18.
Troubleshooting Common Debugging Issues
When things go wrong during development, knowing how to troubleshoot effectively can save hours of frustration. Debugging issues often stem from connection problems, setup errors, or unexpected crashes. By understanding these challenges, we can ensure smoother and more productive sessions.
Resolving Connection and Setup Problems
Connection issues are among the most common challenges developers face. For example, 40% of developers report device recognition problems during remote debugging, often due to USB driver issues19. To resolve this, ensure your device is properly connected and recognized by tools like ADB.
Here are some proven methods to diagnose and fix connection problems:
- Verify USB drivers are installed and up-to-date, especially for Samsung devices20.
- Check the connection mode on your device—switch between “Charge Only” and “Transfer Files” if needed20.
- Use the command ‘adb devices’ to confirm your device is recognized20.
Systematic troubleshooting can prevent wasted time and ensure stable debugging sessions. For instance, 25% of developers recommend switching from WiFi to a wired connection for better stability19.
Tips for Preventing Debugger Crashes
Debugger crashes can disrupt your workflow and delay progress. Common causes include memory overload, incompatible settings, or software conflicts. To minimize these issues, follow these practical tips:
- Limit background processes to reduce memory pressure, a strategy recommended by 65% of developers19.
- Regularly update your development environment to avoid compatibility issues21.
- Use selective logging to monitor critical app behavior without overwhelming the system19.
By implementing these strategies, you can maintain stable and productive debugging sessions. As one developer noted, “Preventing crashes is not just about fixing errors; it’s about creating a reliable development environment.”
Issue | Solution |
---|---|
Device Recognition | Update USB drivers, check connection mode |
Debugger Crashes | Limit background processes, update software |
Connection Stability | Switch to wired connection, use selective logging |
By addressing these common issues, we can streamline our debugging process and focus on delivering high-quality applications. Whether you’re troubleshooting connection problems or preventing crashes, these tips will help you stay productive.
Conclusion
Mastering the art of identifying and resolving issues is essential for delivering high-quality applications. Throughout this guide, we’ve explored both basic and advanced techniques, from setting breakpoints to leveraging tools like ADB and LLDB. These methods not only streamline the process but also enhance app reliability and performance.
Properly setting up developer options and enabling debugging modes on devices is a critical step. Tools like Logcat and Firebase Crashlytics provide real-time insights, helping developers track issues effectively22. Understanding the debugger’s dialog interactions can lead to faster issue resolution, ensuring smoother development workflows.
We encourage you to continually refine your debugging process for more efficient app development. By adopting these practices, you can enhance the reliability and performance of your applications. Remember, effective debugging is not just about fixing errors—it’s about creating a seamless user experience.
FAQ
How do we enable developer options on our device?
What is USB debugging, and why is it important?
Can we debug wirelessly without a USB cable?
How do we use breakpoints in Android Studio?
What are some essential adb commands we should know?
How can we debug native C/C++ code on our device?
What should we do if the debugger crashes frequently?
How do we interpret Logcat output for debugging?
Can we customize debugger configurations for specific needs?
What’s the best way to monitor app performance during debugging?
Source Links
- https://stackoverflow.com/questions/43742552/android-studio-how-to-do-step-by-step-debug-whats-the-shortcut – Android Studio – How to do step-by-step debug? What’s the shortcut?
- https://developer.chrome.com/docs/devtools/remote-debugging – Remote debug Android devices | Chrome DevTools | Chrome for Developers
- https://www.browserstack.com/guide/debugging-tools-in-android – Debugging Tools for Android | BrowserStack
- https://stackoverflow.com/questions/20099444/how-exactly-does-android-debugging-work-and-why-does-my-app-behave-differently-w – How exactly does Android debugging work and why does my app behave differently when debugging it?
- https://www.xda-developers.com/android-developer-options/ – Android Developer Options Explained: Everything you can do with these settings
- https://www.kodeco.com/books/android-debugging-by-tutorials/v1.0/chapters/1-getting-started – Android Debugging by Tutorials, Chapter 1: Getting Started
- https://developer.android.com/studio/debug/dev-options – Configure on-device developer options | Android Studio | Android Developers
- https://stackoverflow.com/questions/4893953/run-install-debug-android-applications-over-wi-fi – Run/install/debug Android applications over Wi-Fi?
- https://developer.android.com/codelabs/basic-android-kotlin-compose-intro-debugger – Use the debugger in Android Studio | Android Developers
- https://google-developer-training.github.io/android-developer-fundamentals-course-concepts-v2/unit-1-get-started/lesson-3-testing,-debugging,-and-using-support-libraries/3-1-c-the-android-studio-debugger/3-1-c-the-android-studio-debugger.html – The Android Studio debugger · GitBook
- https://developer.android.com/tools/adb – Android Debug Bridge (adb) | Android Studio | Android Developers
- https://developer.android.com/studio/debug – Debug your app | Android Studio | Android Developers
- https://medium.com/appcent/advanced-debugging-in-android-part-2-39ba7f7895ef – Advanced debugging in Android (part 2)
- https://www.tothenew.com/blog/advanced-debugging-techniques-in-android-studio/ – Advanced Debugging Techniques in Android Studio | TO THE NEW Blog
- https://codingwithevan.com/mastering-android-debugging/ – Mastering Android Debugging in Android Studio
- https://blog.sentry.io/improve-android-debugging/ – How to Improve Your Android Debugging Process
- https://developer.android.com/topic/performance/measuring-performance – Overview of measuring app performance | App quality | Android Developers
- https://developer.android.com/topic/performance/performance-measurement-examples – Examples of performance measurement and analysis | App quality | Android Developers
- https://www.flexihub.com/android-remote-debugging/ – Android Remote Debugging and Testing
- https://stackoverflow.com/questions/76933014/why-cant-i-debug-my-android-app-on-my-phone – Why can’t I debug my Android app on my phone?
- https://www.dre.vanderbilt.edu/~schmidt/android/android-4.0/out/target/common/docs/doc-comment-check/resources/faq/troubleshooting.html – Troubleshooting | Android Developers
- https://www.zipy.ai/guide/android-debugging – Android Debugging Guide: Tools, Methods, and Common Errors
⭐️ Tap the exclusive deal link https://temu.to/k/uot8tcxvwum to score top-quality items at ultra-low prices. 🛍️ These unbeatable deals are only available here. Shop now and save big! ⭐️ Directly get exclusive deal in Temu app here: https://app.temu.com/m/mhb5rstagbx
Another surprise for you! Click https://temu.to/k/uag0bn0o0wd to earn with me together🤝!